How to program i
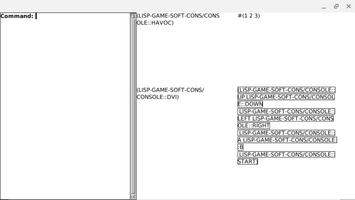
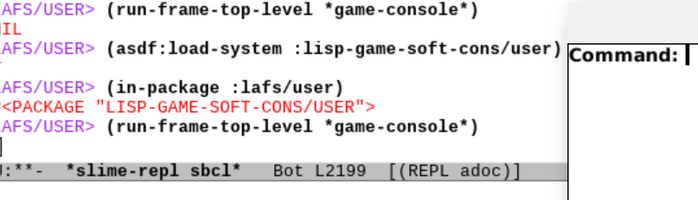
It was a broad answer to what programming is and is like and how to do it.
I'm not just reproducing the video. Since we can move faster in text, we'll do a more gradual example first.
I'm planning a v part series for all of programming there is.
HOW TO PROGRAM i
Table of Contents
- 1. Programming
- 1.1. Common Lisp Object System Language
- 1.1.1. A class in lisp is like this.
- 1.1.2. Classes can have slots
- 1.2. Class from the video
- 1.3. But what's the defpackage above that in the files
- 1.4. Common Lisp Interface Manager
- 1.5. The whole package
- 1.6. The ASD file
- 1.7. Console file
- 1.8. User file
- 1.1. Common Lisp Object System Language
When I made this video https://toobnix.org/w/cYv47BY8FtprzhpHLpiVeY My video wasn't really about lisp, clim, asdf, or a modern software console.
It was a broad answer to what programming is and is like and how to do it.
I'm not just reproducing the video. Since we can move faster in text, we'll do a more gradual example first.
I'm planning a v part series for all of programming there is.
1. Programming
Use lisp. Watch or read one of my introductions to clim slime sbcl emacs if you can't run lisp yet. My 5 minute starter video playlist: https://toobnix.org/w/p/4bRcULzg6bBAyELkRqU6EQ
1.1. Common Lisp Object System Language
1.1.1. A class in lisp is like this.
(defclass whatever-name () ())
#<STANDARD-CLASS LISP-GAME-SOFT-CONS/USER::WHATEVER-NAME>
- Making one of those
(make-instance'whatever-name)
#<WHATEVER-NAME {10063BDC23}>
- class of that
(class-of (make-instance'whatever-name))
#<STANDARD-CLASS LISP-GAME-SOFT-CONS/USER::WHATEVER-NAME>
#<STANDARD-CLASS COMMON-LISP-USER::WHATEVER-NAME>
- class of that
1.1.2. Classes can have slots
A slot of a class has a slot-name and can hold an object in it.
(defclass slotted () ((my-number :initform '8 :reader my-number))) (defvar *slotted* (make-instance 'slotted)) (my-number *slotted*)
8
A reader can be used to get a value but can't be used to set it.
1.2. Class from the video
(defclass game-console-parent () ((game-cartridge :initform '(havoc) :reader cartridge) (chaos-packet :initform #(1 2 3) :reader chaos) (video-display :initform '(dvi) :reader video) (game-controller :initform '(up down left right a b start) :reader controller)))
#<STANDARD-CLASS LISP-GAME-SOFT-CONS/USER::GAME-CONSOLE-PARENT>
1.3. But what's the defpackage above that in the files
Lisp systems use a lisp system making system named ASDF. I use package-inferred-system. This has the rule that every code file starts by defining one special package associated with that file. Then we can forget that files exist because we know what packages are.
(uiop:define-package :lisp-game-soft-cons/console (:mix :clim :clim-lisp :cl) (:export #:game-console #:*game-console*) (:nicknames :lafs/console)) (in-package :lisp-game-soft-cons/console)
It's basically always like this. The default naming is to match the file that defined it.
1.4. Common Lisp Interface Manager
Has one good free software and two major proprietary implementations, and has for more than 35 years. All current.
The spec is a later extension to ANSI common lisp 2e, more or less. I cut down the code a little bit here
(define-application-frame game-console (game-console-parent standard-application-frame) () (:panes (interactor :interactor) (table (tabling (:height 512 :width 512) (list (make-pane :application :display-function 'display-game-cartridge) (make-pane :application :display-function 'display-chaos-packet)) (list (make-pane :application :display-function 'display-video-display) (make-pane :application :display-function 'display-game-controller))))) (:layouts (default (horizontally () interactor table)))) (defun display-game-cartridge (frame pane) (present (cartridge frame) 'expression :stream pane)) (defun display-chaos-packet (frame pane) (present (chaos frame) 'expression :stream pane)) (defun display-video-display (frame pane) (present (video frame) 'expression :stream pane)) (defun display-game-controller (frame pane) (present (controller frame) 'expression :stream pane)) (defvar *game-console* (make-application-frame 'game-console))
I put the functions in special scope which is better in lots of ways.
An interactor is an expert command shell. Table is obviously a 2x2 table of display panes.
1.5. The whole package
It's in =~/common-lisp/lisp-game-soft-cons=
. I'm going to hardcode tangles into there.
1.6. The ASD file
Because I use package-inferred-system, this is very small. I have other examples of putting build instructions for an executable here too, but this is the gist.
(defsystem "lisp-game-soft-cons" :class :package-inferred-system :depends-on (:mcclim :lisp-game-soft-cons/user))
1.7. Console file
It pops up an interactor and four data panels (we made this in the video)
(uiop:define-package :lisp-game-soft-cons/console (:mix :clim :clim-lisp :cl) (:export #:game-console #:*game-console*) (:nicknames :lafs/console)) (in-package :lisp-game-soft-cons/console) (defclass game-console-parent () ((game-cartridge :initform '(havoc) :reader cartridge) (chaos-packet :initform #(1 2 3) :reader chaos) (video-display :initform '(dvi) :reader video) (game-controller :initform '(up down left right a b start) :reader controller))) (define-application-frame game-console (game-console-parent standard-application-frame) () (:panes (interactor :interactor) (table (tabling (:height 512 :width 512) (list (make-pane :application :display-function 'display-game-cartridge) (make-pane :application :display-function 'display-chaos-packet)) (list (make-pane :application :display-function 'display-video-display) (make-pane :application :display-function 'display-game-controller))))) (:layouts (default (horizontally () interactor table)))) (defun display-game-cartridge (frame pane) (present (cartridge frame) 'expression :stream pane)) (defun display-chaos-packet (frame pane) (present (chaos frame) 'expression :stream pane)) (defun display-video-display (frame pane) (present (video frame) 'expression :stream pane)) (defun display-game-controller (frame pane) (present (controller frame) 'expression :stream pane)) (defvar *game-console* (make-application-frame 'game-console))
1.8. User file
Common lisp starts you off in the cl-user package. If your system is meant to be used interactively (and it is meant to be used interactively), you make your own this-package-user package, which mixes all your user-level exports (so they are internal to the user package; your internals are largely invisible).
(uiop:define-package :lisp-game-soft-cons/user (:mix :lisp-game-soft-cons/console :clim :clim-lisp :cl) (:export) (:nicknames :lafs/user)) (in-package :lisp-game-soft-cons/user)
I use the package of /user as a scratch area for common lisp. When it forms into something I give it its own package (file).
Author: SCREWLISP
Created: 2024-06-05 Wed 18:06
Comment here and request how-to devlogs and videos
Follow my mastodon https://mastodon.sdf.org/@screwtape/
Watch my peertube https://toobnix.org/c/screwtape_channel/videos
Files
Get LISP GAME SOFT CONS
LISP GAME SOFT CONS
Forming a committee to spec a lisp game software console
More posts
- Install the-cons, game cartridges using git now plzJun 25, 2024
- Turning Robots Into Life with the LOOP facilityJun 17, 2024
- PAINSTAKING SOURCE COMPARISON vidak's robots vs screwlisp'sJun 16, 2024
- What I learned from example and tictactoeJun 13, 2024
- 40min copy+paste video example-game to make tictactoeJun 13, 2024
- Lisp soft console example game #firstJun 11, 2024
- livecode videos for days. hotloading clim gui deltas.Jun 09, 2024
- Cautionary Tales: Incompatible ECL SFFI SDL2 C/C++ LISPJun 06, 2024
Comments
Log in with itch.io to leave a comment.
itch.io messed a bit with my code in formatting. I will make it work better going forward.